Infrared sensor
Slides
F for fullscreen · O for overview
What is infrared sensor?
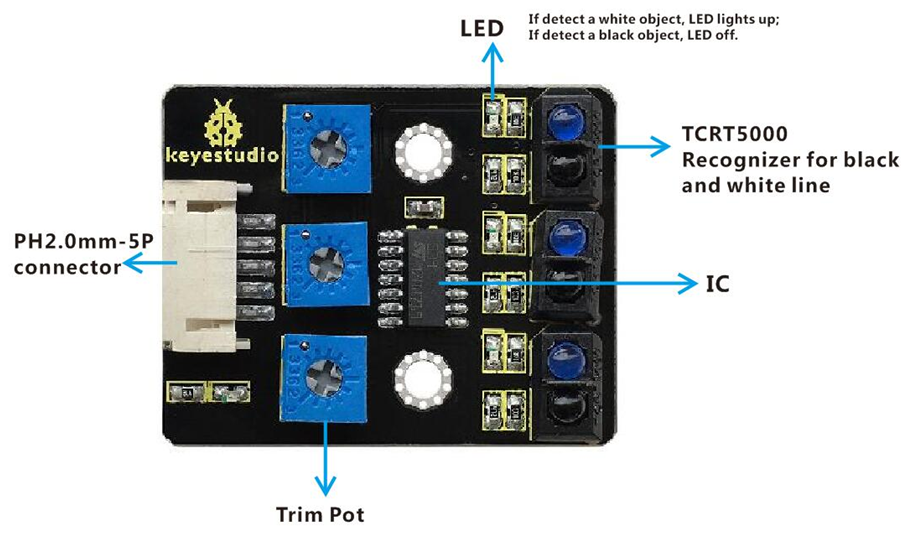

How does infrared sensor work?
Infrared sensors come in pairs of a transmitter and a receiver. The transmitter emits infrared wave/light, and the receiver will receive the infrared wave if the infrared wave is reflected by the surface. If the infrared wave is not reflected by the surface, the receiver will not receive any infrared wave. This is normally used to detect if the surface is white (LOW) or black/dark (HIGH) in colour.
Reading from infrared sensors
Pin mode definition
Purpose | Mode | Pin on board |
---|---|---|
Left sensor | Input | 6 |
Middle sensor | Input | 7 |
Right sensor | Input | 8 |
Light up LED to reflect the state of left sensor
- Define the pin of left sensor as pin D6
- Define LEDpin as Digital 11
- Define the sensor as INPUT
- Define LED as OUTPUT
- Read the state of sensor, if detect the white paper, it is at LOW level
- Light the LED
- Turn off the LED
Light up LED to reflect the state of middle sensor
- Define the pin of middle sensor as pin D7
- Define LEDpin as Digital 11
- Define the sensor as INPUT
- Define LED as OUTPUT
- Read the state of sensor, if detect the white paper, it is at LOW level
- Light the LED
- Turn off the LED
Light up LED to reflect the state of right sensor
- Define the pin of right sensor as pin D8
- Define LEDpin as Digital 11
- Define the sensor as INPUT
- Define LED as OUTPUT
- Read the state of sensor, if detect the white paper, it is at LOW level
- Light the LED
- Turn off the LED
Bring it further
- How do we detect if the robot stays at the middle of a black line on a white background? How about the robot is slightly to the left or right of the black line?