Ultrasonic sensor
Slides
F for fullscreen · O for overview
What is ultrasonic sensor?
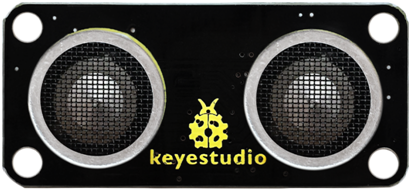
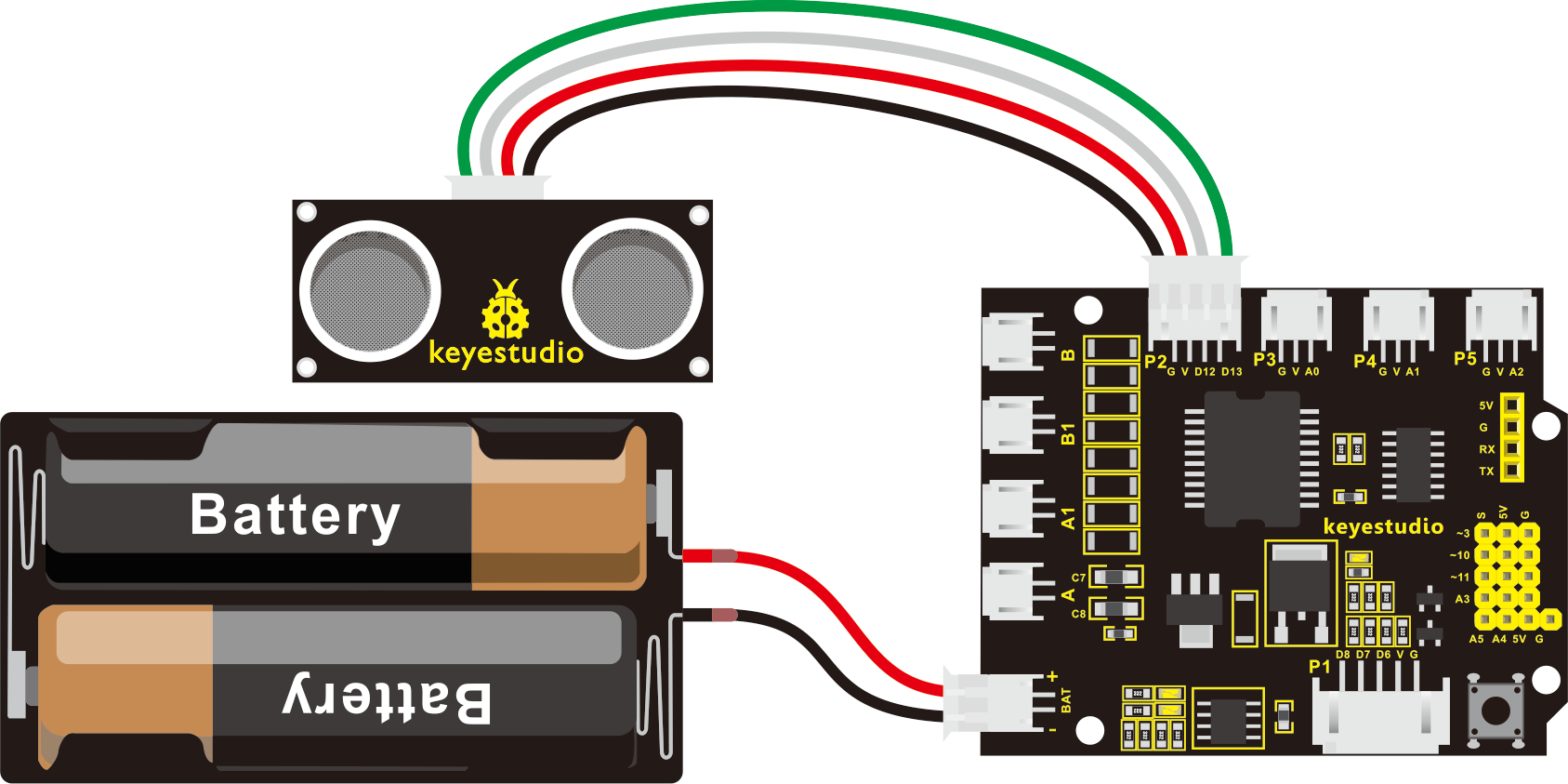
How does ultrasonic sensor work?
Read from an ultrasonic sensor
Pin mode definition
Purpose | Mode | Pin on board |
---|---|---|
Emit sound (Trip) | Output | 12 |
Receive sound (Echo) | Input | 13 |
Distance calculation
Distance of the object in front of the ultrasonic sensor can be calculated using the time between sending and receiving the signal (sound)
Time between sending and receiving = time to travel for \(2d\) = \(T\) µs
Formula derivation
\[\begin{aligned}
\text{distance }d & = \frac{T \text{ µs}\times \text{speed of sound}}{2}\\\\
& = \frac{T \text{ µs} \times 340 \text{ m/s}}{2}\\\\
& = T \times 10^{-6} \text{ s} \times 170 \times 10^2 \text{ cm/s}\\\\
& = 170 \times 10^{-4} \times T \text{ cm}\\\\
& \approx \frac{T}{58} \text{ cm}
\end{aligned}\]
Code implementation
To implement the emission and recipient of sound and calculating the distance of the object in code,
- Emit sound for 12 µs (Start)
- Emit sound for 12 µs (End)
- Receive sound (Start)
- Receive sound (End)
- Calculate distance
Serial monitor to display calculated distance
We can use the serial monitor to display the calculated distance
- Enable serial connection at baud rate of 9600
Serial.print("text")
prints out the textSerial.println("text")
prints out the text and end the line, further printing will start in a new line
Combining the code
int pinTrip = 12;
int pinEcho = 13;
float distance = 0; //(1)
void setup() {
pinMode(pinTrip, OUTPUT);
pinMode(pinEcho, INPUT);
Serial.begin(9600);
}
void loop() {
digitalWrite(pinTrip,LOW);
delayMicroseconds(2);
digitalWrite(pinTrip,HIGH);
delayMicroseconds(12);
digitalWrite(pinTrip,LOW);
distance=pulseIn(pinEcho,HIGH);
delay(10);
distance=distance/58;
Serial.print("distance=");
Serial.print(distance);
Serial.println("cm");
delay(500);
}
- Take note of this line. We need to define the variable to later save our reading from the ultrasonic sensor.
Verify and upload the code to the robot
Launch serial monitor
After the code is running on the robot, launch serial monitor from the Arduino IDE